Pump.Fun Websocket Tutorial - Get A Realtime Token Stream Of Newly Launched Tokens
It's 4 am in the morning and you're smashing the refresh button trying to get in on new tokens as soon as they launch. Wouldn't it be amazing if you could have a bot that gives you pump.fun token addresses as soon as you launch? That's exactly what we're building today!
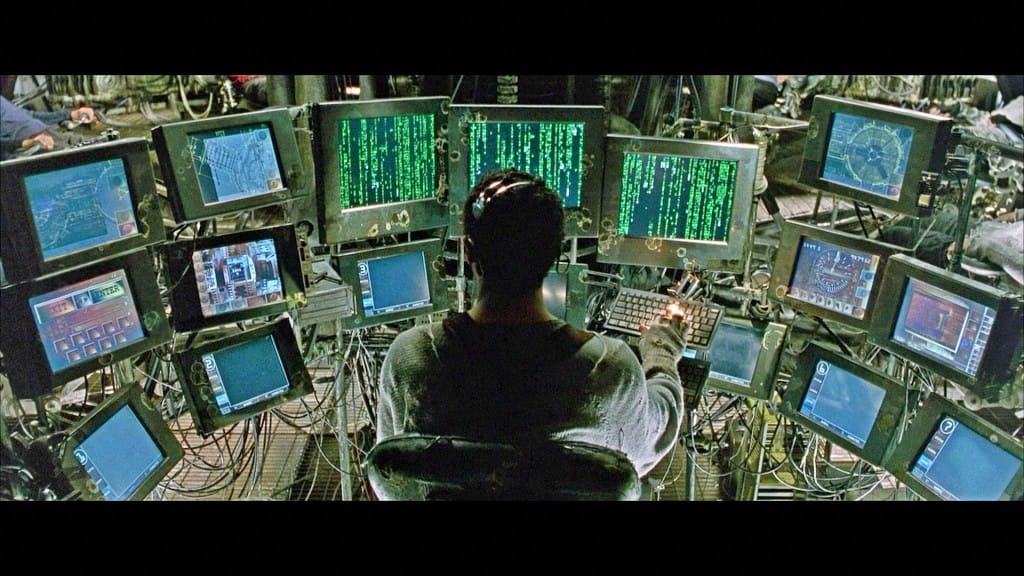
What Do I Need To Start?
- Python Installed (Any Version Is fine - but if you're still using < 2.x, seriously why? Upgrade to 3.x+)
- 10 Minutes (probably just 5 mins if you're into speedrunning).
- Atleast 2 brain cells XD (No scratch that, let's make it 1.)
So What Are We Building?
We're going to build a python app that listens to pump.fun for any tokens that just got created and we're going to print it out on the console. Before we get started, lets get you a free forever api key.
Registering An Account
Head over to nolimitnodes.com and click on Start Building to get an account.
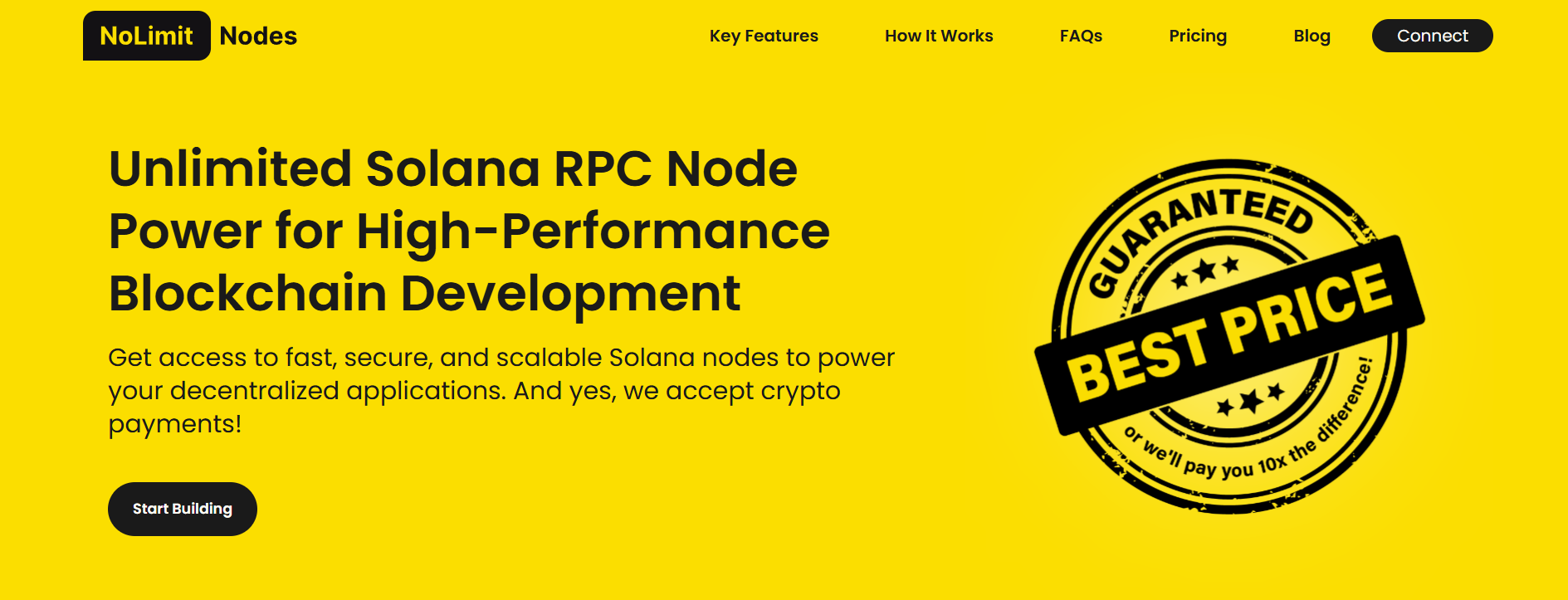
Once you have registered, click on the Get button to get your free API key.
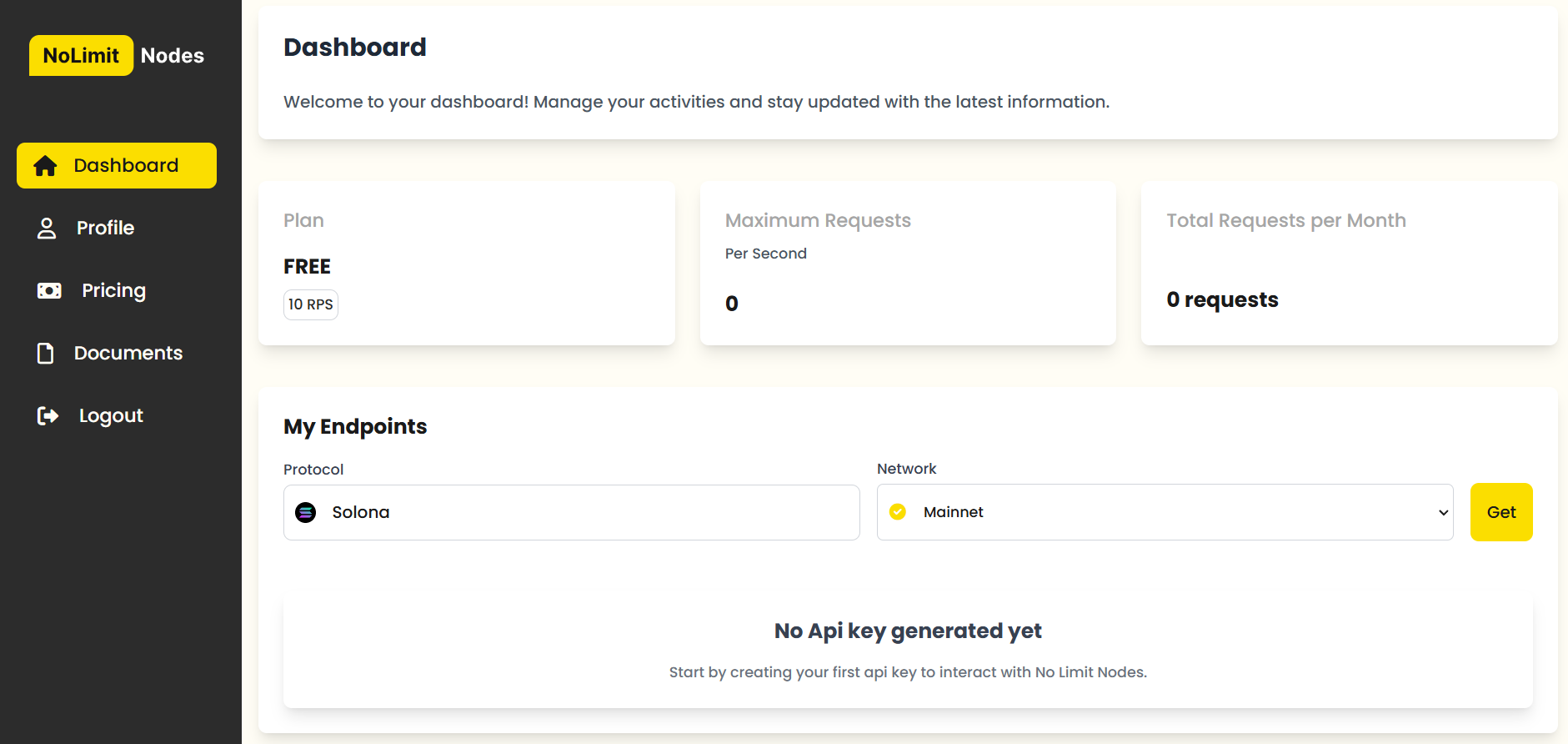
You should then see your generated API
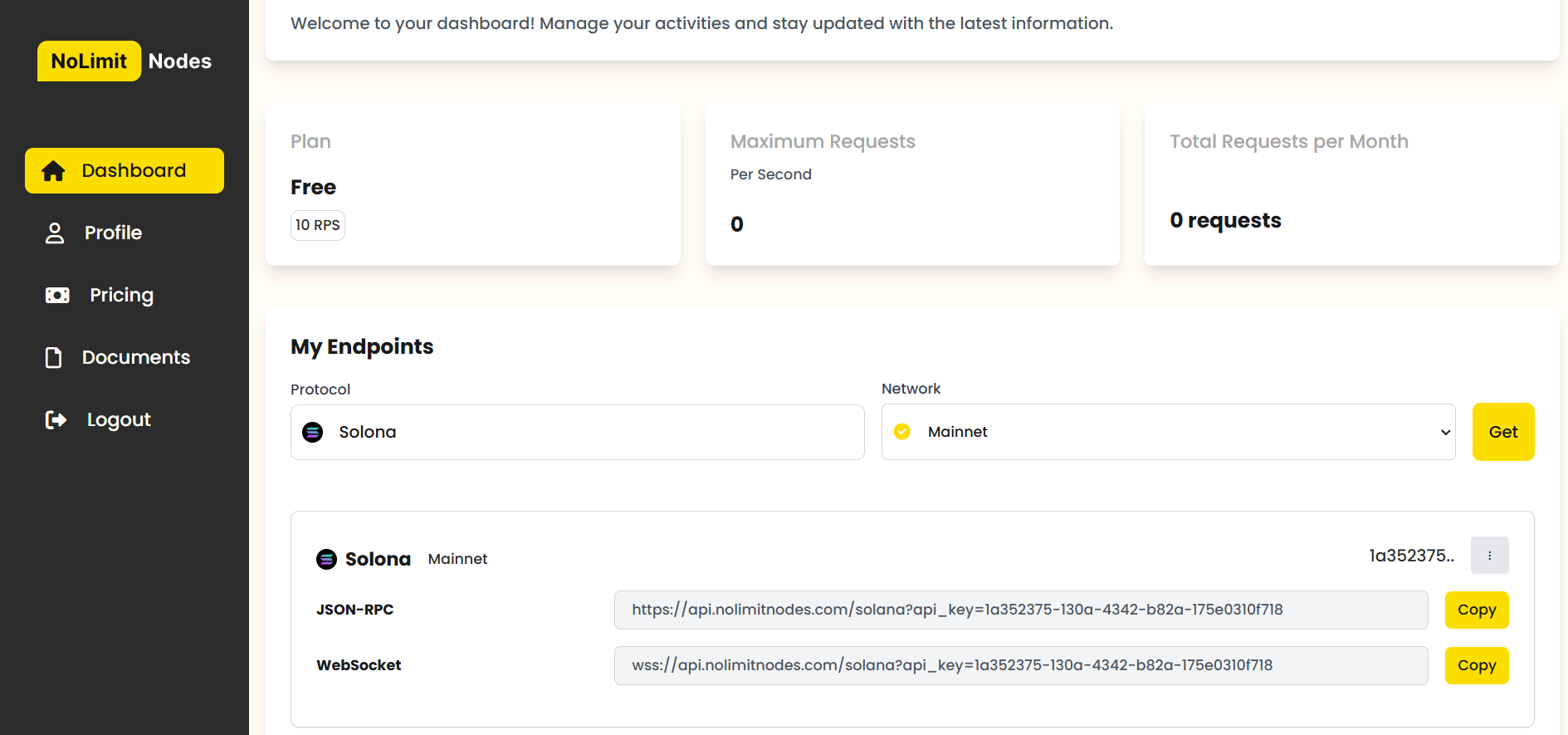
Click copy and take a note of your API key. You will need it for the rest of this tutorial.
Understanding the Structure
Before we get our hands dirty, let's talk about how the websocket api request and the response looks like.
We'll first start by establishing a websocket connection with wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY
Once we have the socket connection open we're going to send this request
{
"method": "pumpFunCreateEventSubscribe",
"params": {
"eventType": "coin",
"referenceId": "litrally-anything-here-to-use-as-reference"
}
}
Once we send this in we'll get a confirmation that the subscription request is a success like this
{
"status": "Create Event Subscribed",
"subscription_id": "9c37a3e8-d39b-497c-902d-162e19a0bcda",
"reference_id": "litrally-anything-here-to-use-as-reference"
}
after the confirmation message, we should keep getting a real-time stream of coins as they get created. We should get coin creation events back in this format.
{
"method": "createEventNotification",
"result": {
"metadata": {
"network": "solana",
"chain": "mainnet-beta",
"block": "308709941"
},
"timestamp": "1734713628",
"name": "TRUMPCOIN",
"symbol": "TRUMPCOIN",
"uri": "https://ipfs.io/ipfs/QmSSme2anrzV4NWrofS3uzXTq9B1L5Jxy54FgvPcrAsrhe",
"mint": "CWVtv9SQMVibEqzFBLy5FZdLhozzZzRDBbX9HGnypump",
"bondingCurve": "HZzaNo92zpqqyTb3pBr5P9fAJ7GT9xnSAxygWLbgUV7X",
"associatedBondingCurve": "BnNAk9AtBvQS3vmv9UpkEoAqPAC96PMUig9HtMdJescU",
"creator_wallet": {
"address": "91U3uKcD2EuC7eW8bbBtC7ftwrNpmgGmJQFpgBZbaBAB"
},
"event_type": "create_coin"
},
"subscription_id": "litrally-anything-here-to-use-as-reference"
}
We see a ton of valuable information in this message. Let me break this down for you.
block
- Gives you the block number when this token was created.timestamp
- Gives you the unix timestamp when this token was created.name
- Gives you the token name.symbol
- Gives you the token's ticker.uri
- Gives you the token's metadata like token image.mint
- Gives you the token's addressbondingCurve
- That's the account that sells/buys from you when you try to buy/sell.associatedBondingCurve
- That's the token account that the bondingCurve uses to store the tokens for this specific token.address
- That's the wallet address of the degen who launched the token.
That's it. that's everything you need to know about the format. Let's get coding.
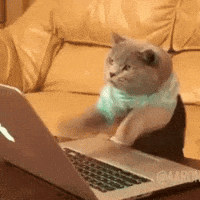
Let's Get Codin'
Fire up your favourite ide (I use PyCharm). Create a new script called token_creation_watcher.py
.
Start by importing websocket and json.
import websocket
import json
Let's send in the websocket request next (dont forget to replace YOUR_API_KEY with your actual api key)
import websocket
import json
socket_url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
ws = websocket.WebSocketApp(
socket_url,
on_open=on_open, # we havent defined this method just yet.
on_message=on_message, # we havent defined this method just yet.
on_close=on_close, # we havent defined this method just yet.
on_error=on_error # we havent defined this method just yet.
)
ws.run_forever()
This is all it takes to establish a websocket connection. Now let's send a subscription message to start getting a stream of newly created tokens.
import websocket
import json
def on_open(ws):
subscribe_message = {
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "1"
}
}
ws.send(json.dumps(subscribe_message))
socket_url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
ws = websocket.WebSocketApp(
socket_url,
on_open=on_open,
on_message=on_message,
on_close=on_close,
on_error=on_error
)
ws.run_forever()
next let's create the on_message method to recieve the coin creation events
import websocket
import json
def on_open(ws):
subscribe_message = {
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "1"
}
}
ws.send(json.dumps(subscribe_message))
def on_message(ws, message):
print("Received data:", json.loads(message))
socket_url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
ws = websocket.WebSocketApp(
socket_url,
on_open=on_open,
on_message=on_message,
on_close=on_close,
on_error=on_error
)
ws.run_forever()
To finish it up we'll also add in the on_close
and the on_error
method to gracefully handle any closing, handling errors. For this tutorial, we'll just print them.
import websocket
import json
def on_open(ws):
subscribe_message = {
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "1"
}
}
ws.send(json.dumps(subscribe_message))
def on_message(ws, message):
print("Received data:", json.loads(message))
def on_close(ws, close_status_code, close_msg):
print("Disconnected from WebSocket")
def on_error(ws, error):
print("WebSocket error:", error)
socket_url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
ws = websocket.WebSocketApp(
socket_url,
on_open=on_open,
on_message=on_message,
on_close=on_close,
on_error=on_error
)
ws.run_forever()
That's it!!!! You're done.
If you want to check the other pump.fun APIs out head over to https://nolimitnodes.com/blog/pump-fun-websocket-build-a-real-time-crypto-trading-bot-with-nolimitnodes-price-data/
If you're stuck anywhere or need general consulting on anything crypto related I'm happy to help. You can email me at robert.king@nolimitnodes.com. I usually check my email once a day.
- Cheers