Pump.Fun WebSocket: Build a Real-Time Crypto Trading Bot with NoLimitNodes Price Data
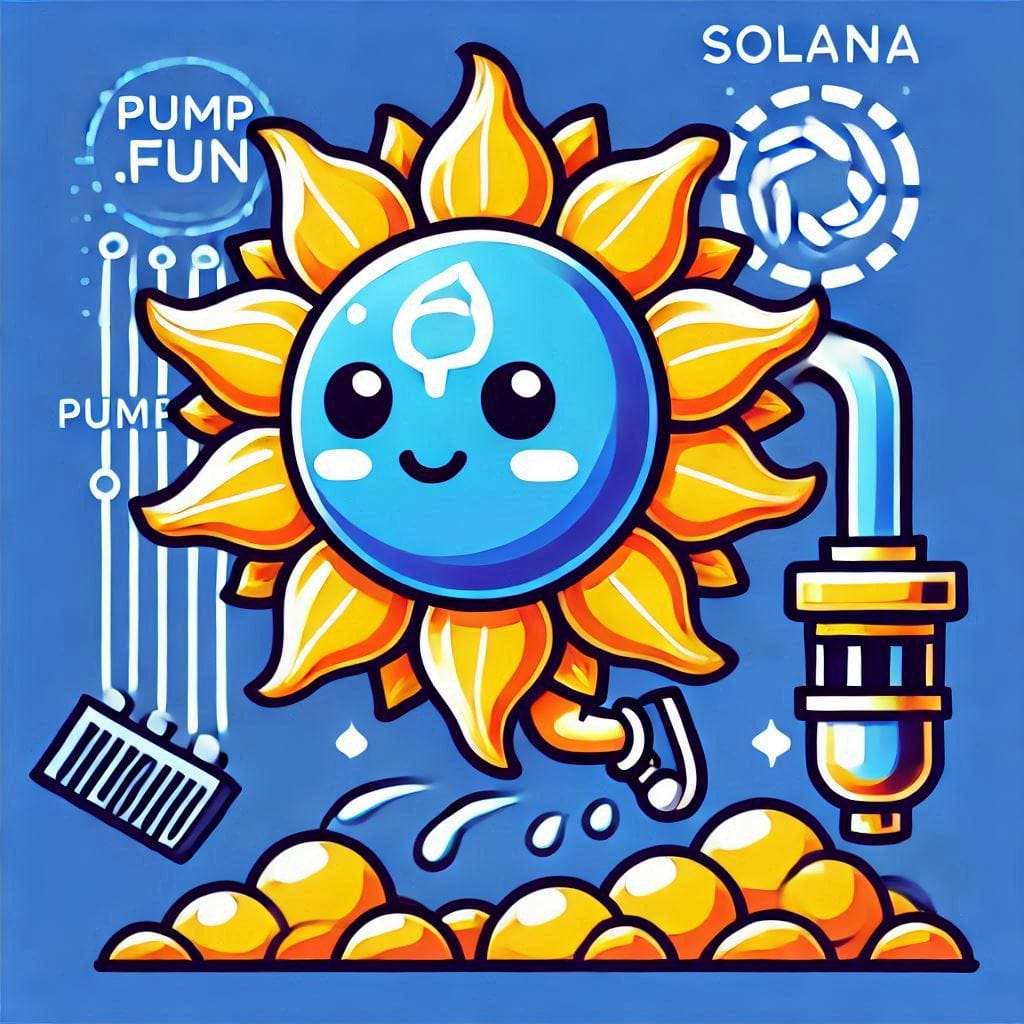
If you're looking to build a killer trading bot to rule over pump.bot, I've got you covered! This quick tutorial is going to do just that. I'm going to show you how to get up and running in 10 minutes.
A Quick History (skip this if you're in a hurry)
Getting access to rich pump.fun data is pretty hard. I wanted to grab pump.fun's api and get some bots going to become a crypto millionare. I looked around for hours to get access to something affordable but found nothing that's useful and easy enough for me to start writing my trading logic.
So I decided to just bite the bullet and use Solana's raw block data to parse it myself and build the bot. After writing thousands of lines of code, I was able to get something that was reliable, stable and easy enough so that I can stop worrying about the pump.fun infrastructure layer and just focus on building an amazing bot!
I shared this code with my Team at NoLimitNodes.com to productize it, so that bot builders like you dont have to go through the same trouble that I did, and focus on what you do best; making killer bots!
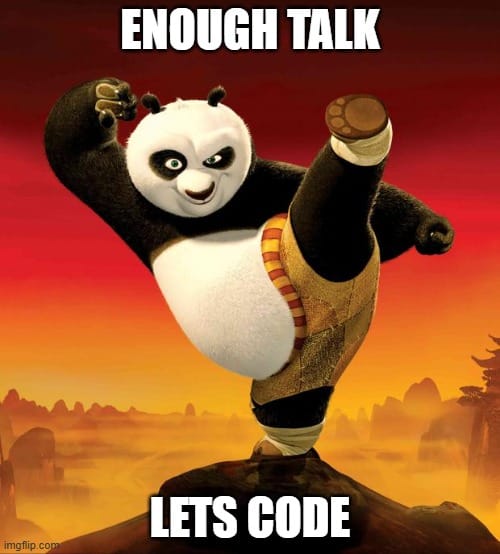
So what do we have?
Your probably asking yourself, "So what kind of data do you guys have?". We give you everything useful that pump.fun emits on the blockchain in a human-readable way. Yes, you read that right, In a human friendly way.

You can get real-time access to
- All tokens as soon as they get created on pump.fun (super useful if you want to be one of the very first people to get in on a coin as it launches)
- All buy/sell transactions along with price data for a single token (when you only want to follow price for 1 token) or for all tokens (if you want all token transaction data for all tokens).
- Token information as soon as it graduates to Raydium.
So its safe to say you've got access to all information that you could possibly need.
Let's start coding by first getting you a 100% free NoLimitNodes Api Key.
Registering An Account
Head over to nolimitnodes.com and click on Start Building to get an account.
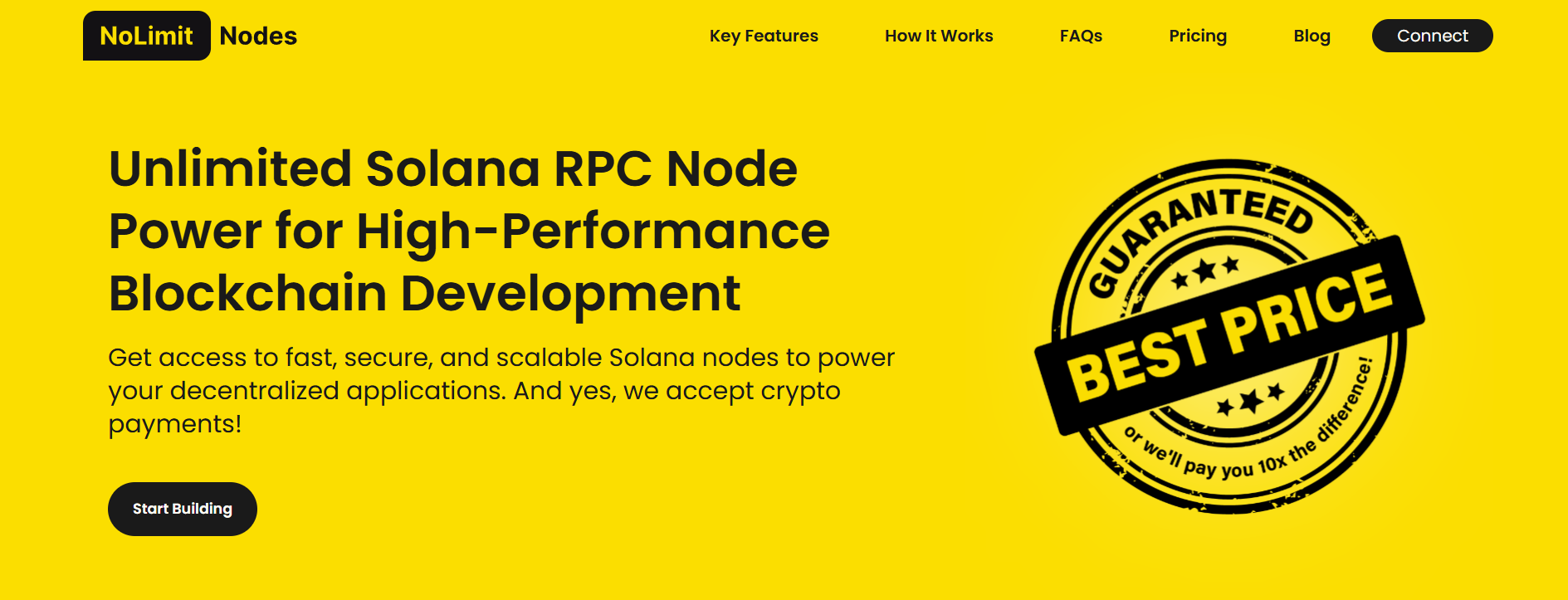
Once you have registered, click on the Get button to get your free API key.
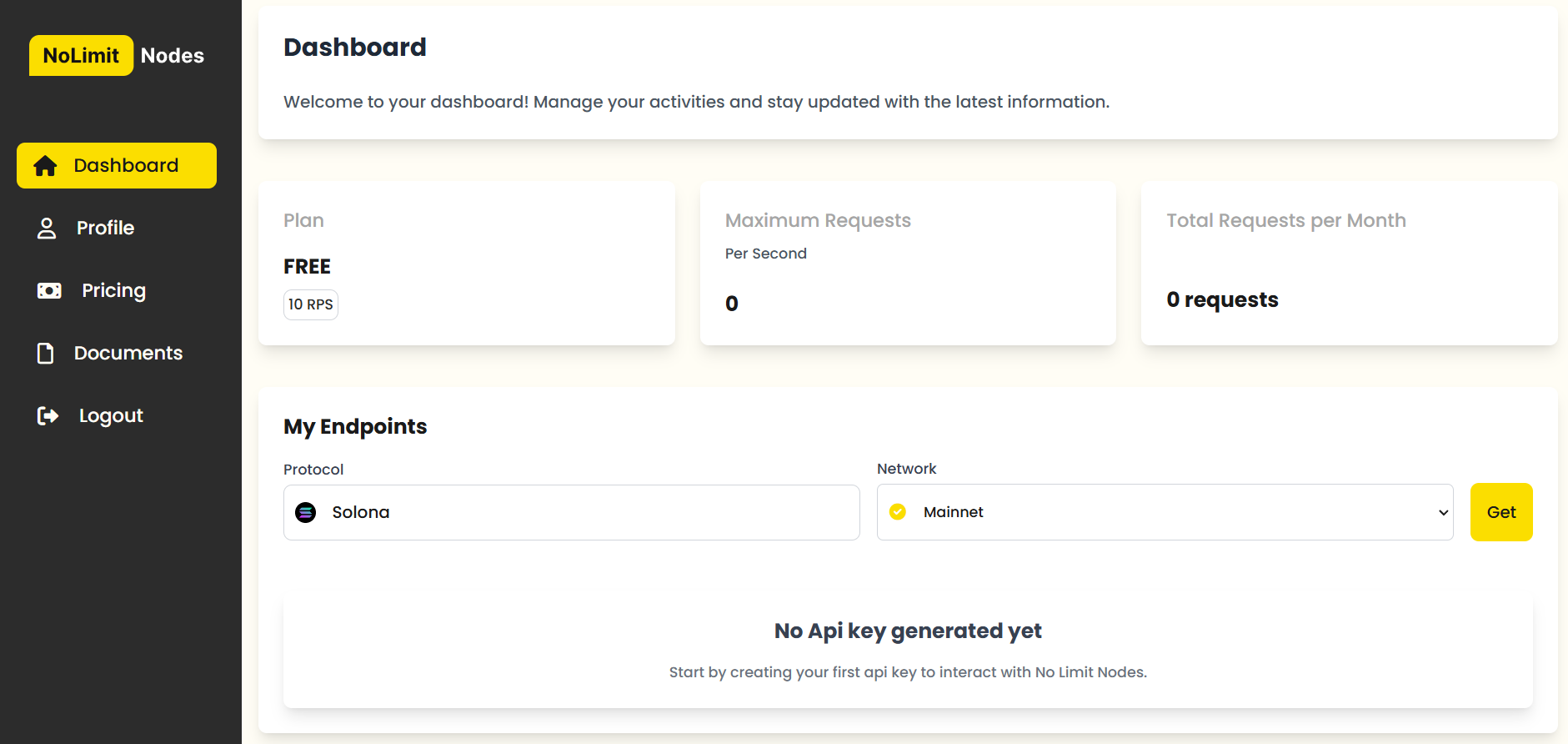
You should then see your generated API
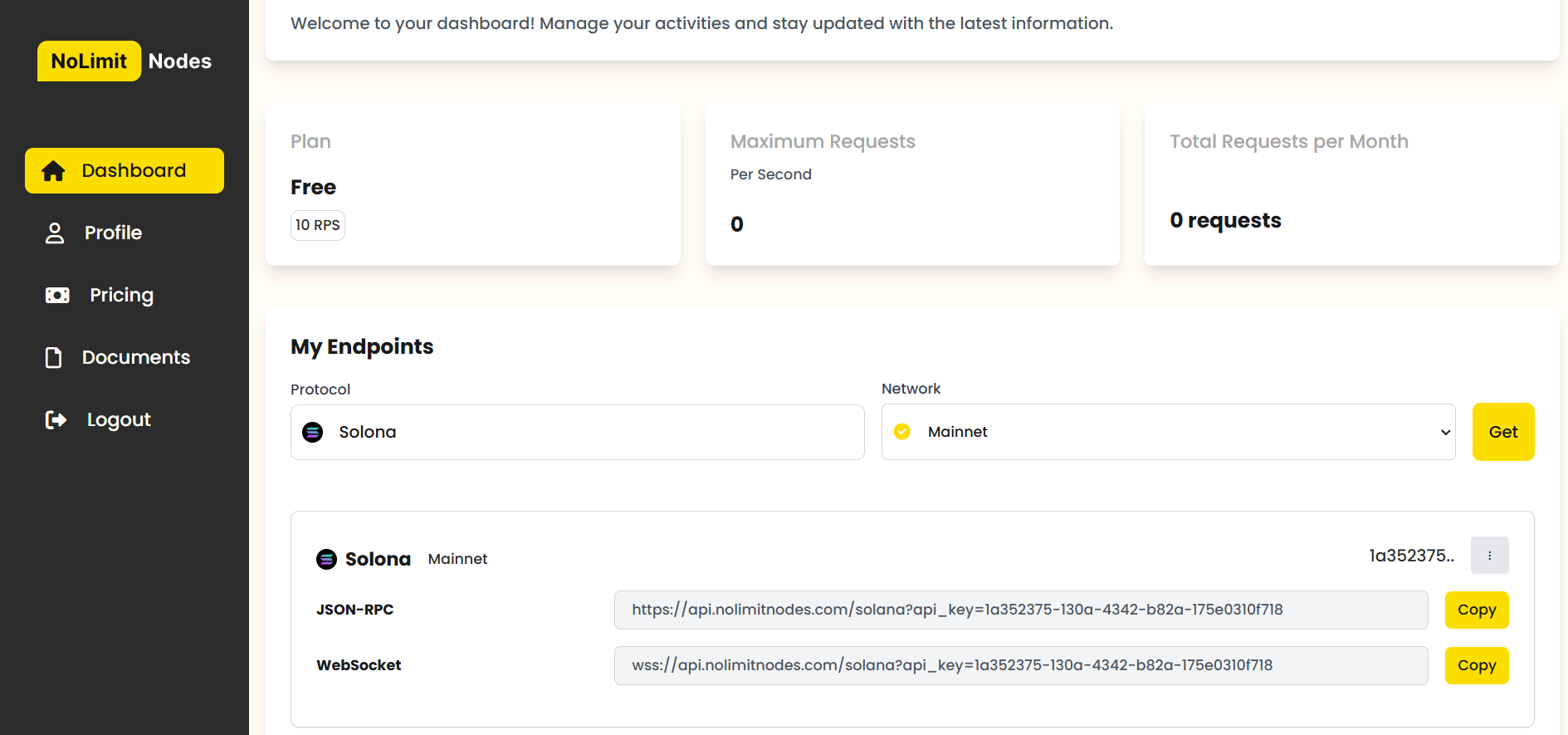
Click copy and take a note of your API key. You will need it for the rest of this tutorial.
Setting Up the Web Socket Connection
Endpoint:
This is how your pump.fun websocket endpoint looks like.
wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY
Replace YOUR_API_KEY
with your API key.
How To Actually Use It
1. Listening to Trade (Buy/Sell) Transactions
Subscribe:
To subscribe to trade transactions for a specific coin:
{
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "abcxxx...pump",
"referenceId": "REF#1"
}
}
To do the same for all coins
{
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "REF#1"
}
}
Note: The referenceId
is simply a string value used to correlate requests with their corresponding responses.
Response:
{
"status": "Trade Subscribed",
"subscription_id": "9d4a3756-f3de-464d-ae05-c36297984f90",
"reference_id": "REF#1"
}
Unsubscribe:
To unsubscribe from trade transactions for a specific coin or all coins:
{
"method": "pumpFunTradeUnsubscribe",
"params": {
"subscriptionId": "9d4a3756-f3de-464d-ae05-c36297984f90"
}
}
Response:
{
"status": "Trade Event Unsubscribed",
"subscription_id": "9d4a3756-f3de-464d-ae05-c36297984f90"
}
2. Listening to Create Events Transactions
Subscribe:
To subscribe to create events for coins or LPs:
{
"method": "pumpFunCreateEventSubscribe",
"params": {
"eventType": "coin" // or "lp" or "all",
"referenceId": "REF#1"
}
}
Response:
{
"status": "Create Event Subscribed",
"subscription_id": "9c37a3e8-d39b-497c-902d-162e19a0bcda",
"reference_id": "REF#1"
}
Note: The referenceId
is simply a string value used to correlate requests with their corresponding responses.
Unsubscribe:
To unsubscribe from create events for coins or LPs:
{
"method": "pumpFunCreateEventUnsubscribe",
"params": {
"subscriptionId": "9c37a3e8-d39b-497c-902d-162e19a0bcda",
}
}
Response:
{
"status": "Create Event Unsubscribed",
"subscription_id": "9c37a3e8-d39b-497c-902d-162e19a0bcda"
}
Example Integration Code
JavaScript Implementation
Here’s how you can integrate the pump.fun WebSocket API in JavaScript:
const WebSocket = require('ws');
const socket = new WebSocket('wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY');
socket.on('open', () => {
console.log('Connected to pump.fun WebSocket');
// Subscribe to trade transactions for all coins
const subscribeMessage = {
method: "pumpFunTradeSubscribe",
params: {
coinAddress: "all",
referenceId: "REF#1"
}
};
socket.send(JSON.stringify(subscribeMessage));
});
socket.on('message', (data) => {
console.log('Received data:', JSON.parse(data));
});
socket.on('close', () => {
console.log('Disconnected from WebSocket');
});
socket.on('error', (error) => {
console.error('WebSocket error:', error);
});
Python Implementation
Here’s how you can integrate the pump.fun WebSocket API in Python:
import websocket
import json
def on_open(ws):
print("Connected to pump.fun WebSocket")
# Subscribe to trade transactions for all coins
subscribe_message = {
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "REF#1"
}
}
ws.send(json.dumps(subscribe_message))
def on_message(ws, message):
print("Received data:", json.loads(message))
def on_close(ws, close_status_code, close_msg):
print("Disconnected from WebSocket")
def on_error(ws, error):
print("WebSocket error:", error)
socket_url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
ws = websocket.WebSocketApp(socket_url,
on_open=on_open,
on_message=on_message,
on_close=on_close,
on_error=on_error)
ws.run_forever()
Key Notes
- Dynamic Coin Selection: Replace
abcxxx...pump
with the specific coin address you want to monitor. Use"all"
to listen to all coins. - Event Type Options:
- For trade transactions, use
pumpFunTradeSubscribe
orpumpFunTradeUnsubscribe
. - For create events, use
pumpFunCreateEventSubscribe
orpumpFunCreateEventUnsubscribe
.
- For trade transactions, use
- Real-Time Updates: The WebSocket connection provides immediate updates for your subscriptions.
Conclusion
The pump.fun WebSocket endpoint from nolimitnodes.com is an efficient way to listen to trade and create events.
This guide provides the foundational steps and example code to help you integrate this powerful API into your applications.
Start leveraging real-time data today and enhance your decentralized application!