Mastering Pump.fun trade with WebSocket Series : Buy , Book profit and set stop loss with NoLimitNodes Data
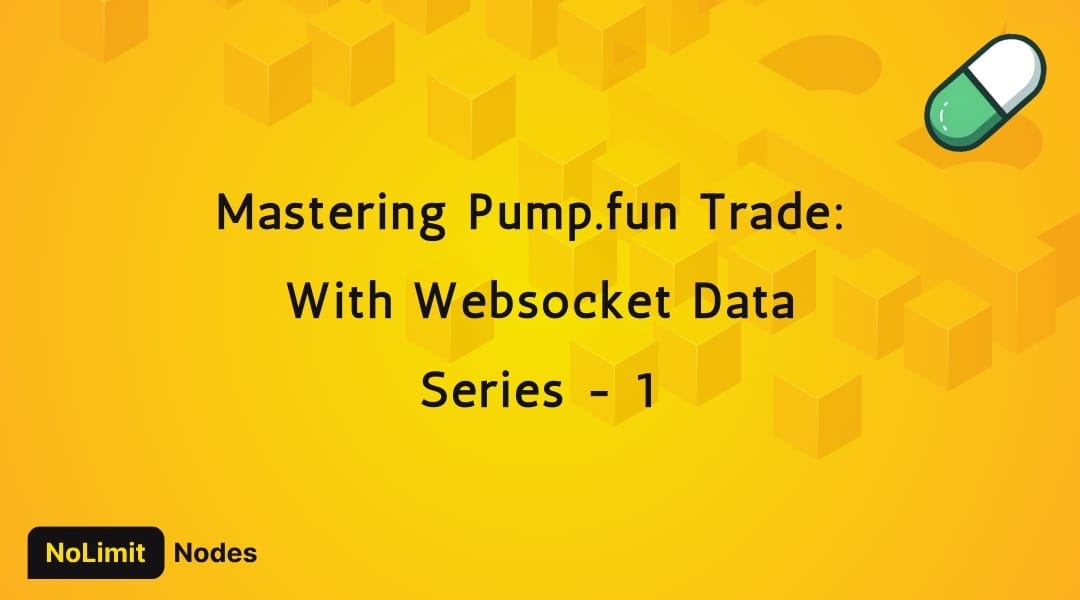
Alright, crypto warriors!
Are you ready to conquer pump.fun with a trading bot so epic it'll blow your mind? Well, hold on tight because I'm about to share the ultimate guide to building a bot that will have you riding the gains wave faster than you can say "To the moon!"
Get ready to level up your crypto game
What Do I Need To Start?
- Python Installed (Any Version Is fine - but if you're still using < 2.x, seriously why? Upgrade to 3.x+)
What Are We Designing Today?
We're going to build an advanced trading bot for the pump.fun platform that leverages WebSocket technology to automate cryptocurrency trading. The bot will monitor new coins and market transactions in real-time, allowing it to execute buy and sell orders based on predefined conditions. With features like notifications for optimal buying and selling points, as well as smart stop-loss mechanisms, this bot aims to maximize profits while minimizing risks. By the end of the guide, you'll have a powerful tool at your disposal that can help you navigate the fast-paced world of crypto trading with confidence!
Registering An Account
Head over to nolimitnodes.com and click on Start Building to get an account.
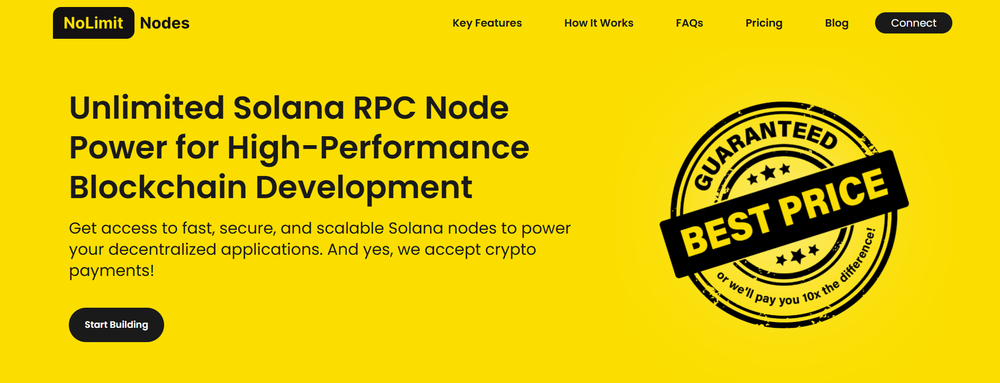
Once you have registered, a token will be generated by default, or you can generate one more by clicking on the Get button to get your free API key.
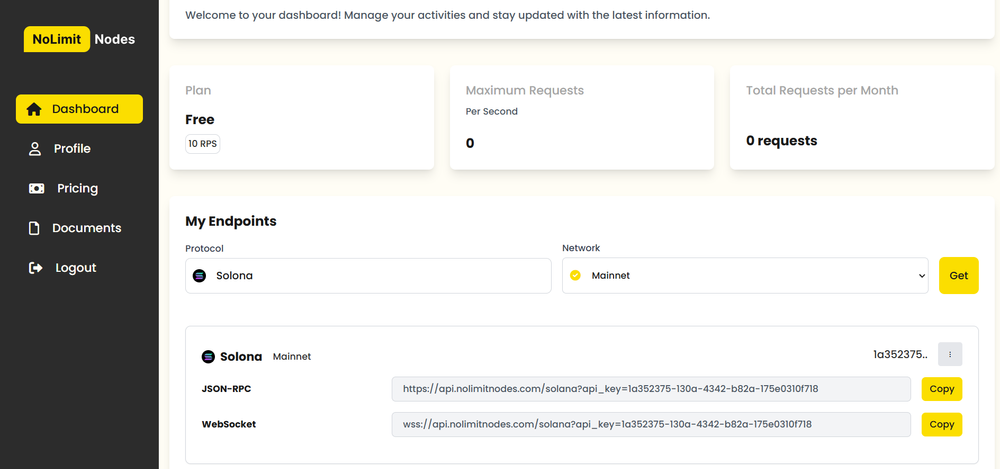
Click copy and take a note of your API key. You will need it for the rest of this tutorial.
Understanding the Structure
Before we get started, let’s walk through how the WebSocket API requests and responses look.
First, we’ll begin by establishing a WebSocket connection using:wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY
Once the socket connection is open, we’ll send this request:
{
"method": "pumpFunCreateEventSubscribe",
"params": {
"eventType": "coin",
"referenceId": "litrally-anything-here-to-use-as-reference"
}
}
After sending this request, we should receive a confirmation that the subscription was successful, like this:
{
"status": "Create Event Subscribed",
"subscription_id": "9c37a3e8-d39b-497c-902d-162e19a0bcda",
"reference_id": "litrally-anything-here-to-use-as-reference"
}
Once confirmed, we’ll continuously receive real-time streams of coins as they are created. The coin creation events come in this format:
{
"method": "createEventNotification",
"result": {
"metadata": {
"network": "solana",
"chain": "mainnet-beta",
"block": "308709941"
},
"timestamp": "1734713628",
"name": "TRUMPCOIN",
"symbol": "TRUMPCOIN",
"uri": "https://ipfs.io/ipfs/QmSSme2anrzV4NWrofS3uzXTq9B1L5Jxy54FgvPcrAsrhe",
"mint": "CWVtv9SQMVibEqzFBLy5FZdLhozzZzRDBbX9HGnypump",
"bondingCurve": "HZzaNo92zpqqyTb3pBr5P9fAJ7GT9xnSAxygWLbgUV7X",
"associatedBondingCurve": "BnNAk9AtBvQS3vmv9UpkEoAqPAC96PMUig9HtMdJescU",
"creator_wallet": {
"address": "91U3uKcD2EuC7eW8bbBtC7ftwrNpmgGmJQFpgBZbaBAB"
},
"event_type": "create_coin"
},
"subscription_id": "litrally-anything-here-to-use-as-reference"
}
Similarly, the WebSocket connection is established for pump.fun transactions. We’ll establish a connection with:wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY
Once the socket connection is open, we’ll send this request to subscribe to trade transactions for a specific coin:
{
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "abcxxx...pump",
"referenceId": "REF#1"
}
}
To subscribe to all coins, we’ll send this request:
{
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "REF#1"
}
}
Note: The referenceId
is simply a string value used to correlate requests with their corresponding responses.
After sending this in, we should receive a confirmation that the subscription request is successful, like this:
Response:
{
"status": "Trade Subscribed",
"subscription_id": "9d4a3756-f3de-464d-ae05-c36297984f90",
"reference_id": "REF#1"
}
Once confirmed, we’ll continuously receive real-time streams of transactions. The tradeEventNotification events come in this format:
{
"method": "tradeEventNotification",
"result": {
"metadata": {
"network": "solana",
"chain": "mainnet-beta",
"block": "308693304"
},
"timestamp": 1734706766,
"type": "Buy",
"price": { "sol": "0.0000000780" },
"token_in": {
"token": "So11111111111111111111111111111111111111112",
"amount": 21340983
},
"token_out": {
"token": "4HTXweoVWfRdXTpvrWCctdED6KUi7Ah1aGq76ubMpump",
"amount": 273799880742
},
"wallet": {
"address": "BNArZsgokea5BxYzhzB9BKqhz7C54fYWkL7CPSPCMP8X"
}
},
"subscription_id": "9d4a3756-f3de-464d-ae05-c36297984f90"
}
Let’s Dive Into Coding
Fire up your favourite ide (I use PyCharm). Create a new script called trading_bot_pumpfun.py
First up, we're importing the libraries that'll give our bot superpowers:
import websocket
import json
import threading
Now, let's set some juicy variables that'll make our bot drool for those sweet, sweet profits:
## Variables
buyPrice = float(0.0000001000) # When we pounce on that crypto goodness
takeProfitPrice = float(0.0000001500) # Cha-ching! Time to cash out
stopLossPrice = float(0.0000000800) # Oops, time to bail!
url1 = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
Next up, we're telling the server exactly what we want. It's like ordering at a drive-thru, but instead of burgers, we're getting hot, fresh crypto data:
# Subscription messages
subscribe_new_coin = {
"method": "pumpFunCreateEventSubscribe",
"params": {
"eventType": "coin",
"referenceId": "hello" # your unique reference id
}
}
subscribe_pump_fun_trade = {
"method": "pumpFunTradeSubscribe",
"params": {
"referenceId": "hello", # your unique reference id
"coinAddress": "all"
}
}
Now, let's set up some smart storage for our potential goldmines:
## Dictionaries to store data
buyDict = {} # Key: mint address, Value: coin details
sellDict = {} # Key: mint address, Value: coin details
Time for the real magic! These functions are gonna make your bot smarter than a whole room full of crypto analysts:
def on_message_new_coin(ws, message):
global buyDict
data = json.loads(message)
if data.get("method") == "createEventNotification":
coin_details = data["result"]
coin_mint = coin_details["mint"]
coin_name = coin_details["name"]
coin_symbol = coin_details["symbol"]
# Add coin to buyDict if it's not already there
if coin_mint not in buyDict:
buyDict[coin_mint] = {
"name": coin_name,
"symbol": coin_symbol,
"details": coin_details
}
def on_message_transaction(ws, message):
global buyDict, sellDict
data = json.loads(message)
if data.get("method") == "tradeEventNotification":
trade_details = data["result"]
trade_token_out = trade_details["token_out"]["token"]
trade_price = float(trade_details["price"]["sol"])
# Handle buy condition
if ((trade_token_out in buyDict) and (trade_price >= buyPrice) and (trade_price < takeProfitPrice)):
coin = buyDict.pop(trade_token_out)
sellDict[trade_token_out] = coin
# print(f"sellDict {sellDict}");
print(f"BUY - Condition met : [{coin['symbol']}] at price [{trade_price:.10f}] (Address: {trade_token_out})")
# Handle sell conditions
else:
if trade_token_out in sellDict:
coin = sellDict[trade_token_out]
# if trade_price > takeProfitPrice or trade_price < stopLossPrice:
if buyPrice <= trade_price < takeProfitPrice:
print(f"SELL - Target Achieved : [{coin['symbol']}] at TakeProfit Price [{trade_price:.10f}] (Address: {trade_token_out})")
del sellDict[trade_token_out]
elif trade_price < stopLossPrice:
print(f"SELL - Stop loss triggered: [{coin['symbol']}] at StopLoss Price [{trade_price:.10f}] (Address: {trade_token_out})")
del sellDict[trade_token_out]
else:
del sellDict[trade_token_out]
def on_error(ws, error):
print(f"Error: {error}")
def on_close(ws, close_status_code, close_msg):
print("WebSocket closed")
def on_open_new_coin(ws):
print("New coin webSocket connection opened")
ws.send(json.dumps(subscribe_new_coin))
print(f"Subscription message sent (WS1): {subscribe_new_coin}")
def on_open_transcation(ws):
print("Transaction webSocket connection opened")
ws.send(json.dumps(subscribe_pump_fun_trade))
print(f"Subscription message sent (WS2): {subscribe_pump_fun_trade}")
Now, let's create a function that'll run our WebSocket connections like a boss:
## Function to create and run a WebSocket connection
def run_websocket(url, on_open, on_message):
ws = websocket.WebSocketApp(
url,
on_message=on_message,
on_error=on_error,
on_close=on_close
)
ws.on_open = on_open
ws.run_forever()
Finally, we're gonna use some threading magic to run multiple WebSockets at once. It's like having a whole team of crypto ninjas working for you 24/7:
## Run both WebSockets in parallel using threading
thread_new_coin = threading.Thread(target=run_websocket, args=(url1, on_open_new_coin, on_message_new_coin))
thread_transaction = threading.Thread(target=run_websocket, args=(url1, on_open_transcation, on_message_transaction))
thread_new_coin.start()
thread_transaction.start()
thread_new_coin.join()
thread_transaction.join()
Want to run the bot and see the magic? Follow these simple steps to get started with Replit:
- Click the "Open on Replit" button provided in the editor below.
- You'll be redirected to the Replit dashboard with the project name "Trading Bot with NolimitNodes."
- Log in to Replit (or sign up if you don’t have an account).
- Click the "Remix this app" button given below the project name.
- Select your project preferences.
- The editor will open with the
main.py
file. - Hit the Run button to see user instructions.
- Check the right corner to find the file name containing the code.
- Don't forget to add your "YOUR_API_KEY"
And there you go! You've successfully set up and run the bot—now sit back and let your crypto ninja do the magic!
Wrapping It Up
That's it, crypto commandos! You've just built a trading bot that's going to make you the talk of the pump.fun town. This bad boy will sniff out new coins, buy them at your designated buy price, and sell them when your take-profit or stop-loss conditions are met.With this bot by your side, you'll be:
- Catching new coins like a pro
- Buying low and selling high on autopilot
- Protecting your gains with smart take-profit and stop-loss strategies
- Laughing all the way to the bank
Remember, with great power comes great responsibility (and potentially great profits ). Use this bot wisely, and may the crypto gods smile upon your trades!
Now go forth and conquer the pump.fun world! And hey, when you're sipping cocktails on your private yacht, don't forget who showed you the way to crypto glory!
What's Next?
Now that you've got the basics down, it's time to dive deeper into advanced strategies—learn how to implement trailing stop-loss to protect your profits and maximize gains! click here https://nolimitnodes.com/blog/mastering-pump-fun-with-websocket-a-tutorial-on-trailing-stop-loss-strategies/
Interested in exploring more of the pump.fun APIs? Head over to https://nolimitnodes.com/blog/pump-fun-websocket-build-a-real-time-crypto-trading-bot-with-nolimitnodes-price-data/ for more details.
If you need assistance or have any crypto-related questions, don't hesitate to contact me. You can email me at robert.king@nolimitnodes.com, and I'll get back to you, usually within a day.