Mastering Pump.fun: Track Real-Time Token Launches, Monitor Trades & Automate Your Strategy with WebSockets
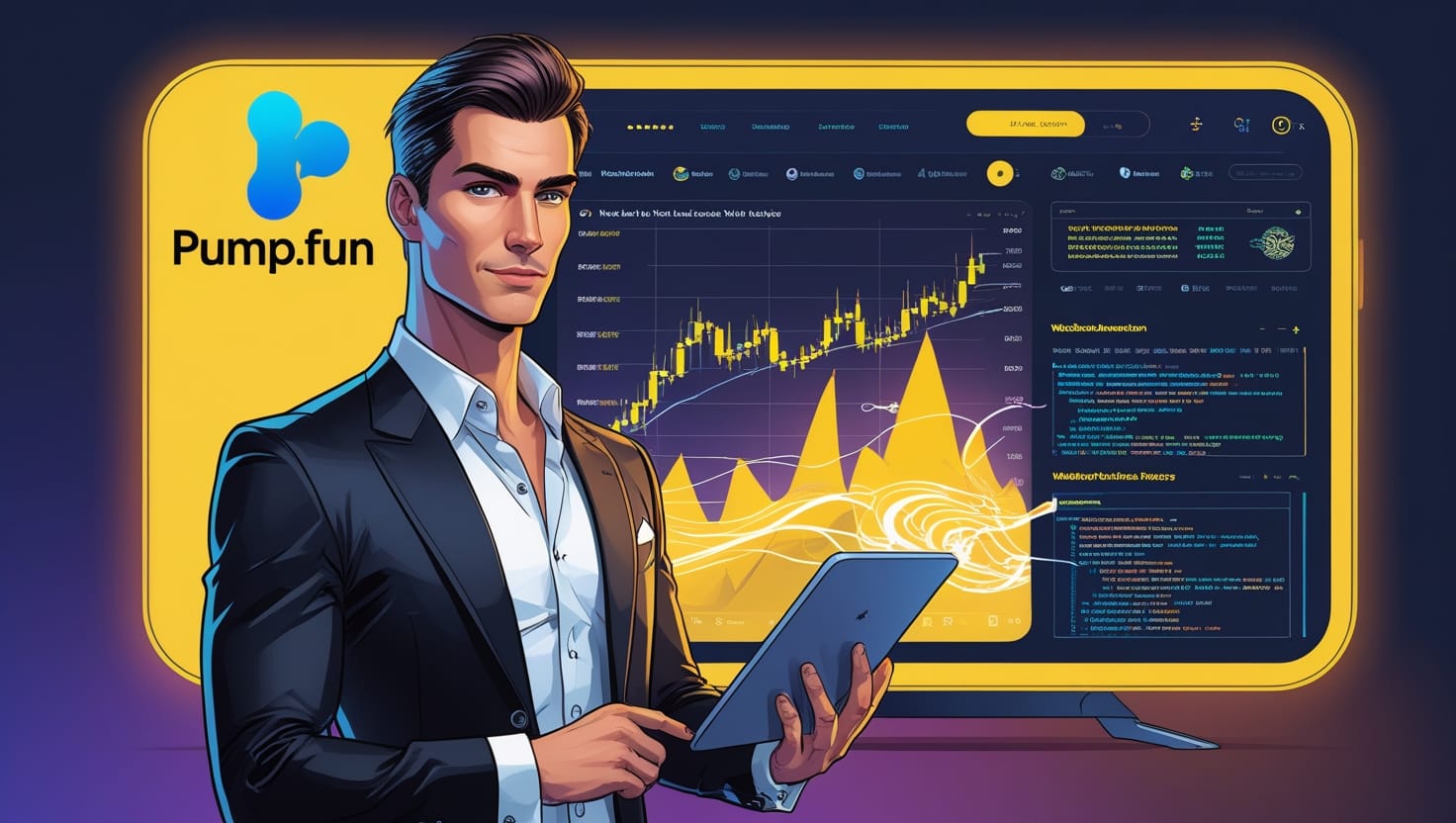
Pump.fun is where chaos meets opportunity. Every few minutes, a new memecoin explodes onto the scene—some destined to moon, others to vanish. But what if you could listen in real time, catch launches early, and automate smart buy/sell moves like a crypto ninja?
That’s exactly what this guide is about.
Whether you’re a degenerate trader, a memecoin explorer, or launching your own coin—this blog takes you from watching to winning on Pump.fun.
Tools You’ll Need
- Python 3.x (upgrade if you haven’t yet)
- Terminal or IDE (VSCode, PyCharm—dealer’s choice)
- A free API Key from NoLimitNodes
What We’re Building
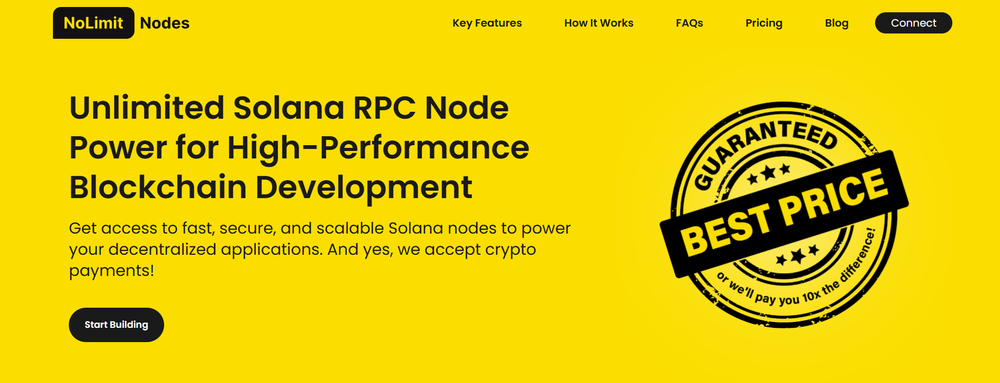
You’ll learn how to:
✅ Listen to new token launches on Pump.fun in real time
✅ Stream live transactions across all Pump.fun memecoins
✅ Automatically buy, take profit, or stop loss using logic
✅ Build the foundation of a trading bot that works while you sleep
How Pump.fun Memecoins Work
Here’s a quick breakdown of the token lifecycle on Pump.fun:
- Token launches with 100% tax — no early flippers.
- As people buy, the tax reduces incrementally.
- When the market cap hits $69K, the token auto-launches on Raydium, and liquidity is burned.
- Early entries can win big—but many tokens never make it to Raydium, aka “rugged”.
Visual Scope:
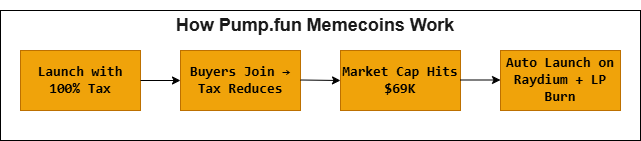
Bonus Insight:
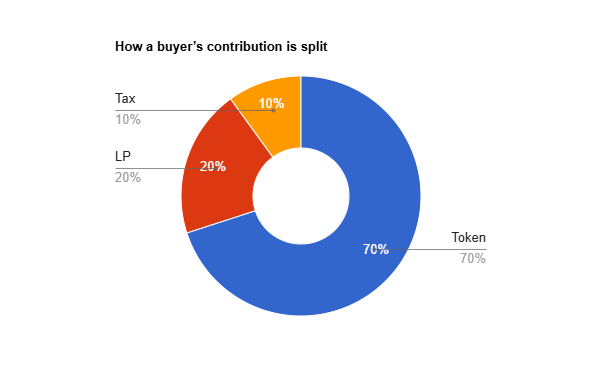
Step 1: Real-Time Launches via WebSocket
Connect to the live token stream:
url = "wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY"
Subscribe to token creation events:
{
"method": "pumpFunCreateEventSubscribe",
"params": {
"eventType": "coin",
"referenceId": "launch-sub"
}
}
This gives you real-time memecoin launches with data like:
- Token name & symbol
- Mint address
- Creator wallet
- IPFS metadata (if available)
Step 2: Listen to All Token Trades
To monitor buy/sell actions across every memecoin, subscribe like this:
{
"method": "pumpFunTradeSubscribe",
"params": {
"coinAddress": "all",
"referenceId": "tx-sub"
}
}
Sample trade notification:
{
"method": "tradeEventNotification",
"result": {
"type": "Buy",
"price": { "sol": "0.000000045" },
"wallet": { "address": "trader-wallet" },
"token_out": { "token": "coinMintAddress", "amount": 10000000 }
}
}
Build Your First Bot
Install the dependency:
bashCopyEditpip install websocket-client
Now paste the following bot script:
import websocket, json, threading
buyPrice = float(0.0000000500)
takeProfitPrice = float(0.0000000900)
stopLossPrice = float(0.0000000300)
buyDict, sellDict = {}, {}
def on_new_coin(ws, msg):
data = json.loads(msg)
if data.get("method") == "createEventNotification":
coin = data["result"]
mint = coin["mint"]
name = coin["name"]
symbol = coin["symbol"]
buyDict[mint] = {"name": name, "symbol": symbol}
print(f"🟢 NEW COIN: {name} ({symbol}) - Mint: {mint}")
def on_trade(ws, msg):
data = json.loads(msg)
if data.get("method") == "tradeEventNotification":
trade = data["result"]
mint = trade["token_out"]["token"]
price = float(trade["price"]["sol"])
if mint in buyDict and buyPrice <= price < takeProfitPrice:
print(f"🛒 BUY: {buyDict[mint]['symbol']} @ {price}")
sellDict[mint] = buyDict.pop(mint)
elif mint in sellDict:
if price >= takeProfitPrice:
print(f"💰 SELL (TP): {sellDict[mint]['symbol']} @ {price}")
del sellDict[mint]
elif price <= stopLossPrice:
print(f"🛑 SELL (SL): {sellDict[mint]['symbol']} @ {price}")
del sellDict[mint]
def run_socket():
ws = websocket.WebSocketApp(
"wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY",
on_message=on_trade
)
ws.on_open = lambda ws: ws.send(json.dumps({
"method": "pumpFunTradeSubscribe",
"params": {"coinAddress": "all", "referenceId": "tx-sub"}
}))
ws.run_forever()
def run_new_coin_socket():
ws = websocket.WebSocketApp(
"wss://api.nolimitnodes.com/pump-fun?api_key=YOUR_API_KEY",
on_message=on_new_coin
)
ws.on_open = lambda ws: ws.send(json.dumps({
"method": "pumpFunCreateEventSubscribe",
"params": {"eventType": "coin", "referenceId": "launch-sub"}
}))
ws.run_forever()
threading.Thread(target=run_socket).start()
threading.Thread(target=run_new_coin_socket).start()
Winning Strategies That Actually Work
- Catch early: Snipe coins under $10k market cap
- Track creators: Favor wallets with past wins
- Set exits: Define TP/SL logic clearly
- Avoid rugs: Check if creator is active on Telegram/X
Is Pump.fun Just Gambling?
Not if you add data + discipline.
Most tokens fail.
Bots help you catch trends, limit loss, and exit before hype dies.
With tools like NoLimitNodes and WebSockets, it’s not just degenerate gambling—it’s calculated chaos.
Bonus Tips for Creators
Planning to launch your own token? Here’s how to stand out:
- Build a Telegram + Twitter presence before launch
- Upload a meme-based litepaper or whitepaper on IPFS
- Highlight real or meme utility in metadata
- Add creator vesting or community wallets for credibility
Useful Links
- NoLimitNodes – Free API & tooling
- Pump.fun – Where memecoins are born
- Raydium – Where tokens go if they make it